Note : Please refresh for new updates and please open in desktop mode in chrome or laptop for full codes ..
15. Write a program to check given year is a leap year or not.
16. Write a program to given value is positive or negative using nested if.
17. Write a program to show student’s performance based on grades using switch case.
OUTPUT :
Enter your grade: a
You got first position in the class.
18. Write a program to check vowel or consonant using switch case.
OUTPUT :
Enter the charater :A
Vowel
19. Write a program to print “Hello World” 10 times on console using while loop.
OUTPUT :
Hello World: 0
Hello World: 1
Hello World: 2
Hello World: 3
Hello World: 4
Hello World: 5
Hello World: 6
Hello World: 7
Hello World: 8
Hello World: 9
20. Write a program to 1 to 10 numbers using while loop.
OUTPUT :
1
2
3
4
5
6
7
8
9
10
21. Write a program to print numbers 1 to 10 using for loop.
OUTPUT :
1
2
3
4
5
6
7
8
9
10
22. Write a program to print numbers 10 to 1 reverse using for loop.
OUTPUT :
10
9
8
7
6
5
4
3
2
1
23. Write a program to 10 to 1 numbers reverse using while loop.
OUTPUT :
10
9
8
7
6
5
4
3
2
1
24. Write a program to find all odd or even numbers till 50 using for loop.
OUTPUT :
Even num is:: 2
Even num is:: 4
Even num is:: 6
Even num is:: 8
Even num is:: 10
Even num is:: 12
Even num is:: 14
Even num is:: 16
Even num is:: 18
Even num is:: 20
Even num is:: 22
Even num is:: 24
Even num is:: 26
Even num is:: 28
Even num is:: 30
Even num is:: 32
Even num is:: 34
Even num is:: 36
Even num is:: 38
Even num is:: 40
Even num is:: 42
Even num is:: 44
Even num is:: 46
Even num is:: 48
Even num is:: 50
Odd num is:: 1
Odd num is:: 3
Odd num is:: 5
Odd num is:: 7
Odd num is:: 9
Odd num is:: 11
Odd num is:: 13
Odd num is:: 15
Odd num is:: 17
Odd num is:: 19
Odd num is:: 21
Odd num is:: 23
Odd num is:: 25
Odd num is:: 27
Odd num is:: 29
Odd num is:: 31
Odd num is:: 33
Odd num is:: 35
Odd num is:: 37
Odd num is:: 39
Odd num is:: 41
Odd num is:: 43
Odd num is:: 45
Odd num is:: 47
Odd num is:: 49
25. Write a program to break a loop.
OUTPUT:
1
2
3
4
26. Write a program to use skip a value of loop by using continue data type.
OUTPUT :
1
2
3
4
6
7
8
9
10
27. Write a program to print the first value of 1d array.
OUTPUT :
Array first indexing value: 9
28. Write a program to print the 1d array’s values on console.
OUTPUT :
Array arr[0] values: 9
Array arr[1] values: 3
Array arr[2] values: 5
29. Write a program to create 2d array and print all the array’s values on console.
OUTPUT :
Array arr[0][0] values: 1
Array arr[0][1] values: 2
Array arr[0][2] values: 3
Array arr[1][0] values: 2
Array arr[1][1] values: 3
Array arr[1][2] values: 4
Array arr[2][0] values: 3
Array arr[2][1] values: 4
Array arr[2][2] values: 5
Array arr[3][0] values: 4
Array arr[3][1] values: 5
Array arr[3][2] values: 6
30. Write program to print any value of 2d array on console.
OUTPUT :
Array arr[1][2] values: 4
31. Write a program to add two numbers using functions.
OUTPUT :
Enter the two numbers for sum: 15 32
Sum of two numbers: 47
32. Write a program to find factorial of a number by making a function.
OUTPUT :
Enter the numbers which you want factorial: 5
factorial of 5 number : 120
33. Write a program to swap two numbers by making a function.
OUTPUT :
Enter first number:
12
Enter second number:
32
Before Swapping
First number: 12
Second number: 32
After Swapping
First number: 32
Second number: 12
34. Write a program to calculate area of a square using function.
35. Write a program to calculate the average of five numbers using function without return value.
OUTPUT:
Enter the five no to find the average:8 4 9 4 2
average of the 5 no:5.400000
36. Write a program to check whether a number is even or odd using function with return value and argument.
OUTPUT:
Enter the num:37
num is : odd
37. Write a program to adding value in variable using function and call by value.
OUTPUT:
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=100
38. Write a program to swap two numbers using function and call by value.
OUTPUT:
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 10, b = 20
39. Write a program to adding value in variable using function and call by reference.
OUTPUT :
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=200
40. Write a program to swap two numbers using function and call by reference.
OUTPUT :
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 20, b = 10
41. Write a program to find maximum number between two numbers using function.
OUTPUT :
Enter two numbers: 14
14
Both are equal
42. Write a program to find given number’s factorial using function recursion.
OUTPUT:
Enter the number:7
5040
43. Write a program to print the address and value of a variable using pointer.
OUTPUT :
Pointer Example Program : Print Pointer Address
[a ]:Value of A = 10
[*pt]:Value of A = 10
[&a ]:Address of A = 0061FF1C
[pt ]:Address of A = 0061FF1C
[&pt]:Address of pt = 0061FF18
[pt ]:Value of pt = 0061FF1C
44. Pointer Program to swap two numbers without using the 3rd variable.
OUTPUT :
Before swap a:5,b:4
After swap a:4,b:5
45. Write a program to print address and value of two variables using double pointers.
OUTPUT :
Value of var = 789
Value of var using single pointer = 789
Value of var using double pointer = 789
46. Write a program to make string array and print the array’s values.
OUTPUT :
Enter a String: CODEWITHRAVIKANT
CODEWITHRAVIKANT
47. Write a program to copy to string, add two string and find the length of string using string’s functions.
OUTPUT:
add two string in result:hello
add two string in result: hellohello
print length of result string:10
48. Write a program to make two book structures book 1 and book 2, where structures have title, author, subject and id just copy values of these to book 2 structure and print them.
Output:
Id:123
Tittle:Coding
Subject:C-Programming
Author:Dennis Ritchie
49. Write a program to make a union.
OUTPUT:
The value stored in member1 = 15
50. Write a program to create&read a file.
OUTPUT:
This file is open now
Hello world
51. Write a program to write to a file.
OUTPUT:
This file is open now
52. Write a program check a number if Armstrong number.
OUTPUT:
enter the number=153
armstrong number
53. Write a program to make a Fibonacci series.
OUTPUT:
Enter the number of elements:15
0 1 1 2 3 5 8 13 21 34 55 89 144 233 377
54. Write a program to draw basic graphics construction like line, circle, arc, ellipse and rectangle.
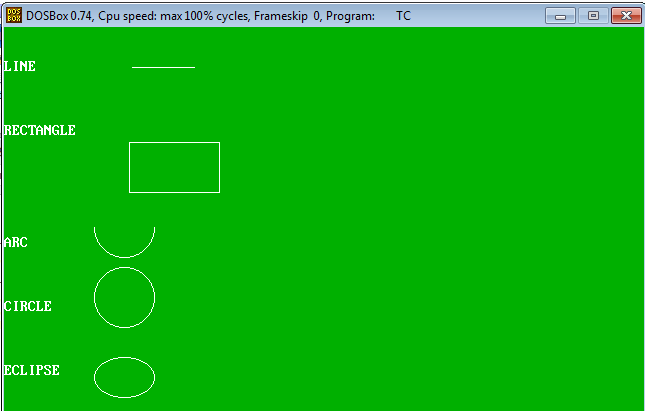